Code reviews in open source communities can be particularly tricky. There may be dozens, hundreds, or even thousands of contributors to a project maintained by a much smaller group of developers; one of the biggest challenges open source maintainers face is triaging and responding to incoming contributions. This challenge is compounded by the fact that open source contributors come from diverse backgrounds, and they may not always be familiar with project requirements, coding standards and norms, or what to expect from the code review process. This disconnect between maintainer needs and contributor knowledge can cause disruptions that bog down the process and worsen the experience for everyone.
Challenges like this are why we built gitStream, a workflow automation tool that enables you to use YAML configuration files to optimize your code review process. It helps add context to PRs, assign code reviews, and automate the merge process. Here are eight ways open source communities can automate the code review process with gitStream.
Welcome First Time Contributors
New contributors are the lifeblood of open source communities; today’s newbie could be tomorrow’s trusted leader. Your first interaction with newcomers sets the standard for all future interactions, so you want to get started on the right foot. gitStream can help by posting welcome messages to first-time contributors that set expectations about the process moving forward and where to go if they want to learn more.
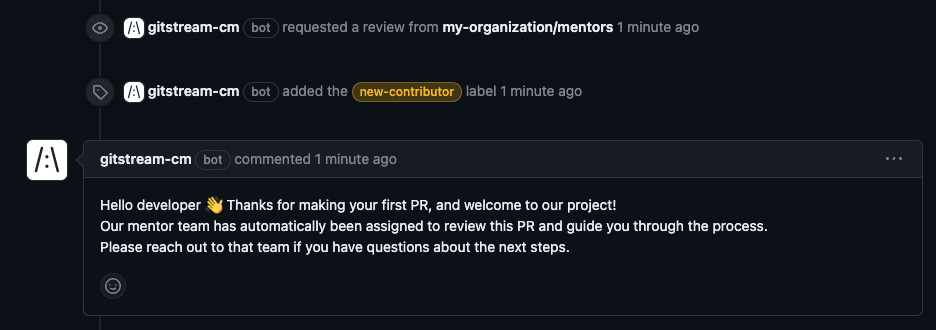
gitStream can also label PRs from first time contributors to indicate that the PR might need more attention. Better yet, if you have a team dedicated to mentoring new contributors, you can assign them as PR reviewers.
# -*- mode: yaml -*-
manifest:
version: 1.0
automations:
# Help newcomers find mentors to guide them.
first_time_contributor:
# If the PR author made their first contribution on the current day
if:
- {{ repo.author_age < 1 }}
# 1. Add reviewers from the team `my_organization/mentors`. Replace this string to match your organization and team
# 2. Apply a new-contributor label.
# 3 Post a comment that explains the next steps.
run:
- action: add-reviewers@v1
args:
reviewers: [my_organization/mentors]
- action: add-label@v1
args:
label: 'New Contributor'
color: 'colors.green'
- action : add-comment@v1
args:
comment: |
Hello {{ pr.author }} 👋 Thanks for making your first PR, and welcome to our project!
Our mentor team has automatically been assigned to review this PR and guide you through the process.
Please reach out to that team if you have questions about the next steps.
colors:
green: '0e8a16'
Assign Code Experts for Review
You might already be familiar with CODEOWNERS as a valuable tool to set basic rules for assigning reviewers based on files and directories. gitStream can also do this, but it goes further with a few key capabilities.
First, gitStream can look all the way down to the function level to detect deprecated components and automatically request changes to the PR. Second, gitStream also can identify code experts and automatically suggest them for code reviews.
This automation will automatically recommend PR reviewers based on the highest level of code expertise whenever someone applies a 'Suggest Reviewer' label to the PR.
# -*- mode: yaml -*-
manifest:
version: 1.0
automations:
assign_code_experts:
# Triggered when someone applies a suggest-reviewer label to a PR.
if:
- {{ pr.labels | match(term='suggest-reviewer') | some }}
# More info about code experts
# https://docs.gitstream.cm/filter-functions/#codeexperts
run:
- action: explain-code-experts@v1
args:
gt: 10
Alternatively, If your community seeks to grow and distribute maintainer knowledge, it’s also straightforward to configure gitStream to distribute code reviews across multiple people to help expand project knowledge horizontally. This next example distributes review responsibilities across individuals with previous experience with the affected portions of the code.
automations:
share_knowledge:
if:
- {{ pr.labels | match(term='Share Knowledge') | some }}
run:
- action: add-reviewers@v1
args:
reviewers: {{ repo | codeExperts(gt=30, lt=60) | random }}
- action: add-comment@v1
args:
comment: |
gitStream has assigned a reviewer to increase knowledge sharing on this PR.
gitStream makes it easy to set custom rules for review assignment that meet the needs of diverse situations, such as when you want to apply extra scrutiny to sensitive code, or create reviewer watch lists for specific resources in your project.
Estimate Time to Review
gitStream can estimate the number of minutes it would take to review a PR, giving maintainers a better sense of which PRs to prioritize based on availability. This helps reduce the mental burden of code reviews by assisting you in identifying quick wins vs. complex changes that require you to plan for focus time to tackle them.
Here’s an example of how to use gitStream to label PRs with an estimated review time. This will automatically apply a color-coded label to every PR that indicates how many minutes it should require someone to review it.
# -*- mode: yaml -*-
manifest:
version: 1.0
automations:
estimated_time_to_review:
if:
- true
run:
- action: add-label@v1
args:
label: "{{ calc.etr }} min review"
color: {{ colors.red if (calc.etr >= 20) else ( colors.yellow if (calc.etr >= 5) else colors.green ) }}
calc:
etr: {{ branch | estimatedReviewTime }}
colors:
red: 'b60205'
yellow: 'fbca04'
green: '0e8a16'
Label PRs by Code Changes
PR titles rarely illustrate the scope of the changes entirely. Labels that indicate the relative volume of changes to various parts of your code base can help maintainers quickly decipher which PRs most apply to them.

The next example shows how to use gitStream to label the percentage of changed code by project directories and resources:
# -*- mode: yaml -*-
manifest:
version: 1.0
automations:
{% for item in labels %}
label_resource_percent_{{ item.name }}:
if:
- {{ files | match(list=item.resources) | some }}
run:
- action: add-label@v1
args:
label: '{{ item.additions | round }}% {{ item.name }}'
{% endfor %}
resources:
core:
- src/app
- src/core
mobile:
- src/android
- src/ios
docs:
- docs/
labels:
- name: Core
resources: {{ resources.core }}
additions: {{ branch.diff.files_metadata | filter(attr='file', list=resources.core ) | map(attr='additions') | sum / total.additions * 100 }}
- name: Mobile
resources: {{ resources.mobile }}
additions: {{ branch.diff.files_metadata | filter(attr='file', list=resources.mobile ) | map(attr='additions') | sum / total.additions * 100 }}
- name: Docs
resources: {{ resources.docs }}
additions: {{ branch.diff.files_metadata | filter(attr='file', list=resources.docs ) | map(attr='additions') | sum / total.additions * 100 }}
total:
additions: {{ branch.diff.files_metadata | map(attr='additions') | sum }}
Approve and Merge Dependabot Updates
Dependabot seems nearly universal for open source projects to keep their software more secure by automatically submitting PRs for dependency updates. gitStream enables you to go one step further to automatically approve and merge Depdendabot updates so your code always stays ahead of CVEs:
# -*- mode: yaml -*-
manifest:
version: 1.0
automations:
approve_dependabot:
if:
- {{ branch.name | includes(term="dependabot") }}
- {{ branch.author | includes(term="dependabot") }}
run:
- action: approve@v1
- action: add-label@v1
args:
label: "approved-dependabot"
- action: merge@v1
args:
wait_for_all_checks: true
squash_on_merge: true
Check out the gitStream integrations page for details about how to use gitStream with languages, frameworks, security tools, other software development services.
Verify Test Coverage
Test coverage often feels like a necessary evil, but it makes a massive difference if your goal is to build reliable software. It takes a village to develop and maintain adequate test coverage, and it can often fall through the cracks due to a lack of awareness or familiarity with the project requirements.
gitStream makes it easy to flag contributions that contain new classes or objects but lack required tests.
# -*- mode: yaml -*-
manifest:
version: 1.0
automations:
label_prs_without_tests:
if:
- {{ files | match(regex=r/[^a-zA-Z0-9](spec|test|tests)[^a-zA-Z0-9]/) | nope }}
run:
- action: add-label@v1
args:
label: 'missing-tests'
color: '#E94637'
Enforce Copyright Headers
If you take open source license compliance seriously, you might have a policy that requires copyright headers on all source code files in your repository. This example shows how to use gitStream to check for copyright headers in all new source code files.
# -*- mode: yaml -*-
manifest:
version: 1.0
automations:
enforce_copyright_header:
if:
- {{ source.diff.files | filter(attr='new_file', regex=r/src\//) | map(attr='original_file') | match(regex=r/^$/) | some }}
- {{ source.diff.files | matchDiffLines(regex=licence.licenceRegex) | nope }}
run:
- action: add-comment@v1
args:
comment: |
All new files in the '/src' directory must include the required copyright header at the top of the file. For example:
// Copyright (c) ORG and contributors. All rights reserved.
// Licensed under the MIT license. See LICENSE file in the project root for details.
licence:
licenceRegex: r/(Copyright \(c\) )|(Licensed under the MIT license)/
Provide Review Tips
One last use case for gitStream that demonstrates the breadth of gitStreams customizability is how it can provide contextual information for any type of PR review. For example, gitStream can recommend helpful labels the contributor can apply or flag issues with security alerts, missing project tracker information, missing data objects, and more.
Get Started
Head over to the docs to learn how to get started with gitStream; you can have your first automation up and running in as little as two minutes. If you want to propose a new automation example, make a feature request, or report an issue, join us on GitHub. We'd also appreciate you giving the repo a star to help us spread the word!