Bugs, logical mistakes, resource-draining variables. Whatever form they might take, finding the bugs hidden in a snippet of code can be tricky. But the quality of your code can affect much more than just whether it works or not. We won’t delve into the fiddly details here – we’ve already written about why code quality is important. But the higher the quality – the more elegant and concise it is – the fewer errors you’ll encounter, the less time you’ll have to spend fixing them, and faster you can make your development cycles.
In this post, we’re going to look at the tools you can leverage to raise the quality of the code your team ships.
Table of Contents
- What Is “Low Quality” Code?
- The Top Five Static Code Analysis Tools
- How to Pick Your Dynamic Analysis Tools
- The Top Five Peer Code Review Tools
- Code Quality v. Quantity: The False Dichotomy
- Conclusion
What Is “Low Quality” Code?
Before we start thinking about the solutions, let’s take a moment to better understand the problem. When we say that a piece of code is bad, what do we mean exactly?
We could mean that the code contains an error – something obviously wrong that violates a style rule or will cause undesirable behavior. There are two main types of errors:
- Syntax errors: These are analogous to writing mistakes: misspelled words, incorrectly placed characters, extra spaces, etc. For these, you’ll want a static code analysis tool, specifically a linter. More on these later.
- Logical errors: These are much harder to spot. It’s easy for a piece of software to scan code for misplaced commas or extra spaces; it’s much harder for a piece of software to understand the logic you’re trying to implement in code. For this, a dynamic analysis tool, which analyzes your code as it runs inside a virtual environment.
But “low quality” doesn’t just refer to how many errors a piece of code contains. Code that is free of errors can still be low quality. So what other dimensions factor into code quality? There are six main ones:
- Readability – How easy is it for someone else to read and understand your code?
- Flexibility – How easy is it for your code to be adapted?
- Reusability – How easy is it for your code to be reused?
- Scalability – How easy can your code be scaled up to more users?
- Extendability – How easily can your code be built upon?
- Maintainability – How easy is it to fix bugs in your code and keep it up to date as other parts of the codebase change?
Notice the common theme: Easy. Is a piece of code easy to work with? This is a key part of determining code quality, but it is also subjective, making it hard to evaluate programmatically. That’s why you need code reviews.
Now that we’ve defined “low quality code,” let’s look at the tools you can use to improve code quality.
The Top Five Static Code Analysis Tools
Static analysis refers to the process of analyzing code that is not running. The main advantage of static analysis is that it can happen quickly, often even in real-time, because it doesn’t require the application to be running.
There are a plethora of static analysis tools, and many of them are built on open source code or are entirely open source. They typically integrate with your CI/CD tools, like GitLab, Jenkins, Bamboo or TeamCity.
With so many options, it’s hard to know which ones to pick. Here are the ones I recommend:
- SonarQube: Probably the most popular static code analysis tool out there. It can scan through 29 different languages and can check your source code before you make a pull request. The biggest benefit of SonarQube is that it checks through your code as you write. It also has a “quality gate,” which blocks any code that doesn’t reach a certain quality threshold from going into production. There is an open source version as well as paid versions.
- Codacy: While this tool doesn’t check as you write, it does check every time you make a pull request, so you can still make sure your code is up to par before it gets pushed live. While it’s perhaps not as common as SonarQube, it does have more favorable reviews.
- DeepSource: A popular tool, though somewhat limited. Its main drawback is that it doesn’t work with as many programming languages. However, in February this year they released a beta version for .NET languages. As they expand, it could easily rise in the ranks.
- Embold: Another tool that’s worth checking out. It scans your whole codebase to give you metrics and identify areas for improvement like security weak points and anti-patterns.
- Veracode: This one is primarily marketed as a way to improve your application security and find holes in your software. It’s a very thorough tool that will definitely improve your code, but the scans can take quite a bit of time. As such, it’s best to use it as a final check to make sure that you’ve not left in any vulnerabilities.
How to Pick Your Dynamic Analysis Tools
Dynamic analysis tools examine your application while it is running in a virtual environment. This can reveal issues that static analysis never could, like never-ending recursion or performance bottlenecks.
Picking the right dynamic analysis tool is tricky because they tend to be language-specific and use-case-specific. Thankfully, there’s a community-maintained list on GitHub broken down by language. Once you narrow down the language, then you can find a tool that can analyze your code for the aspects that you care about.
The Top Five Peer Code Review Tools
A core ethos of software development is to automate whenever possible. Static and dynamic analyses are valuable because they can automatically detect issues that reduce the quality of your code. But they won’t catch everything.
The biggest limitation with static and dynamic analysis is that they can’t check your business logic. They don’t know the functionality you’re trying to implement, so they can’t tell you whether your code is going to behave as expected.
For this, you need another developer. That’s where code reviews come in. By giving another person the opportunity to review your code, they can catch issues with the business logic but also ways to make your code cleaner that the analysis tools didn’t catch.
Developers are often reluctant to do code reviews because they’re time-consuming. And they’re right – they do take time. But they are incredibly valuable.
According to Smartbear’s State of Software Quality report, code reviews are the top thing that a company can do to improve their code quality. Since 2018, over a fifth of the people they surveyed put it as their first priority.

Because code reviews are so valuable, and time-consuming, it is crucial to adopt the right tools to make them easier and more effective. Here are some of the best tools out there today:
- SmartBear Collaborator: This tool helps you work together on code from anywhere. You can review code, sign off on changes, and plug into pretty much any Source Code Manager. It is a bit pricey, though – prices start at over $500 a year.
- Crucible: The stand-out feature is perhaps its inline reviewing. You can see the entire thread of conversation connected to the specific lines of code. It essentially turns your code comments into proper discussions. Very useful. Another benefit is that it’s a one-off payment, though it can get quite pricey for larger companies.
- Review Board: This is a very different way of working from the other examples. Rather than having discussions connected to the code, you instead upload a file to review. In some ways, this can be helpful: You can review any document. But in others, it can be rather restricting. But it’s free, which is always a bonus.
- Gerrit: A free and open source tool, Gerrit is a useful way to work, if you’re using Git. It’s definitely worth checking out, and it can help if you’re on a shoestring budget. But it can be a little slow.
- LinearB: Not to toot our own horn here – but one of the reasons we built LinearB was to help us do code reviews better. In LinearB, you can track key code review metrics, like how long reviews are taking as well as how thorough they are. With our WorkerB automation bot, you can set up notifications to be alerted when a delta was merged without or a review has maybe been forgotten about.
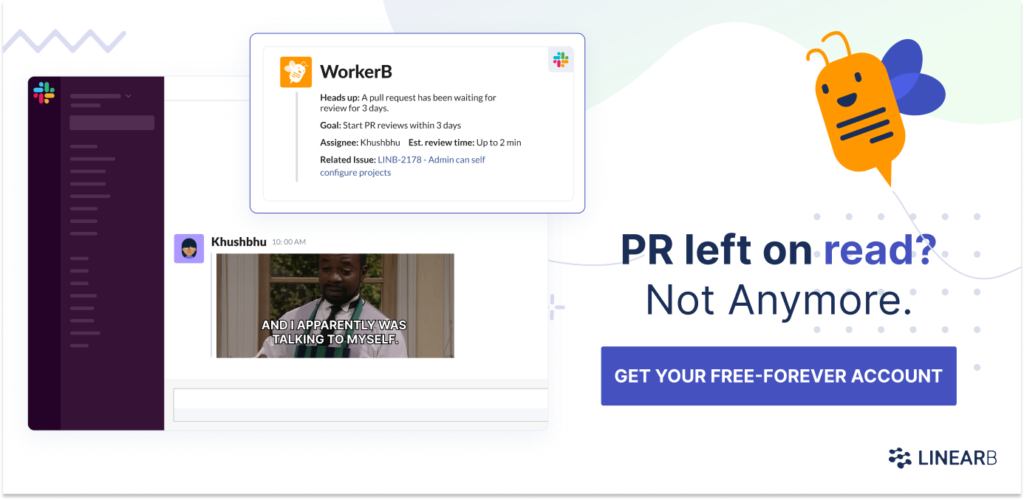
Code Quality v. Quantity: The False Dichotomy
In many areas of life, there appears to be an inherent trade-off between quality and quantity: You can go faster and sacrifice quality or you can more slowly and have higher quality.
This also applies in software development – especially in the continuous integration / continuous deployment (CI/CD) paradigm where speed is so important. But it only applies to an extent. Sacrificing quality for speed is how you accrue technical debt. But by improving quality you actually make it easier to go faster in the future. Remember: One of the chief reasons that makes code low quality is that it is hard to work with, meaning it is hard to write more code on top of it.
This counterintuitive relationship between quality and quantity is what LinearB’s Co-Founder and CEO, Ori Keren, calls the Bicycle Syndrome. He explained on the Dev Interrupted podcast:
Conclusion
If the quality of your code is low, the quality of your application is going to be low as well. In an evermore competitive business landscape, it is paramount to move quickly, but it is also essential to make sure that your codebase is still high quality so that it is secure, reliable, performant, and easy to build upon.
There are a number of tools you can use to raise the quality of code your team ships. In addition to improving your code review process, LinearB can boost code quality in a number of other ways as well, which you can learn more about on our code quality solutions page.
LinearB is all about helping you optimize your development processes so that you can ship better code faster. Our Pipeline Metrics help you to identify inefficiencies. From there, our Team Goals help you set goals and track your progress toward them, and our WorkerB automation bot helps you hit those goals. Finally, our Project Delivery Tracker gives you a holistic view of the work your development teams are putting in and how this connects with the larger business context, enabling you to foster alignment, plan more accurately, and deliver more value.
To learn how easy it is to start leveraging LinearB and how powerful it can be, reach out to set up a demo.
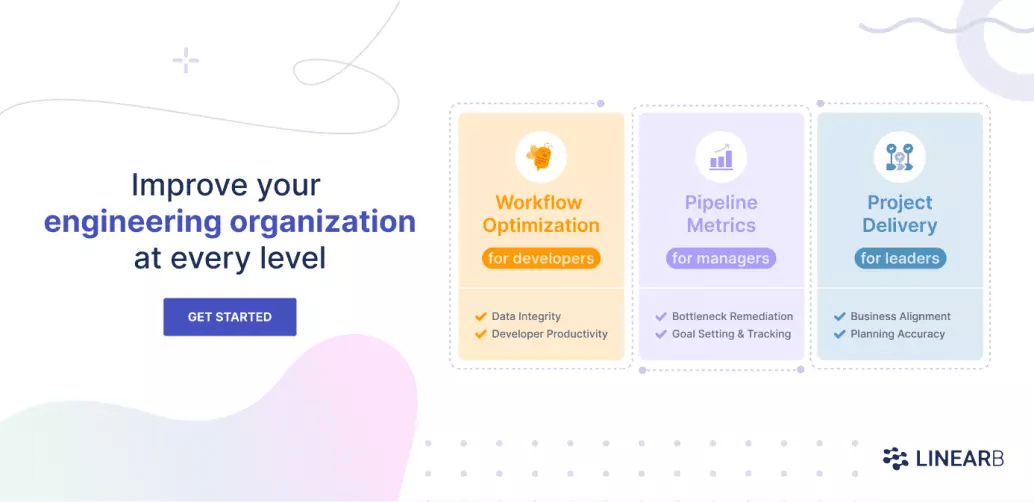